This repository has been archived by the owner on Mar 1, 2024. It is now read-only.
-
Notifications
You must be signed in to change notification settings - Fork 736
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
5 changed files
with
254 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
# Bing Search Tool | ||
|
||
This tool connects to a Bing account and allows an Agent to perform searches for news, images and videos. | ||
|
||
You will need to set up a search key using Azure,learn more here: https://learn.microsoft.com/en-us/bing/search-apis/bing-web-search/overview | ||
|
||
## Usage | ||
|
||
This tool has more a extensive example usage documented in a Jupyter notebook [here](https://github.com/emptycrown/llama-hub/tree/main/llama_hub/tools/notebooks/bing_search.ipynb) | ||
|
||
Here's an example usage of the BingSearchToolSpec. | ||
|
||
```python | ||
from llama_hub.tools.google_search.base import BingSearchToolSpec | ||
from llama_index.agent import OpenAIAgent | ||
|
||
tool_spec = BingSearchToolSpec(api_key='your-key') | ||
|
||
agent = OpenAIAgent.from_tools(tool_spec.to_tool_list()) | ||
|
||
agent.chat('whats the latest news about superconductors') | ||
agent.chat('what does lk-99 look like') | ||
agent.chat('is there any videos of it levitating') | ||
``` | ||
|
||
`bing_news_search`: Search for news results related to a query | ||
`bing_image_search`: Search for images related to a query | ||
`bing_video_search`: Search for videos related to a query | ||
|
||
This loader is designed to be used as a way to load data as a Tool in a Agent. See [here](https://github.com/emptycrown/llama-hub/tree/main) for examples. | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1 @@ | ||
## init |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,66 @@ | ||
"""Bing Search tool spec.""" | ||
|
||
from llama_index.tools.tool_spec.base import BaseToolSpec | ||
from typing import Optional, List | ||
import requests | ||
|
||
ENDPOINT_BASE_URL = 'https://api.bing.microsoft.com/v7.0/' | ||
|
||
class BingSearchToolSpec(BaseToolSpec): | ||
"""Bing Search tool spec.""" | ||
|
||
spec_functions = ["bing_news_search", "bing_image_search", "bing_video_search"] | ||
|
||
def __init__(self, api_key: str, lang: Optional[str] = 'en-US', results: Optional[int] = 3) -> None: | ||
"""Initialize with parameters.""" | ||
self.api_key = api_key | ||
self.lang = lang | ||
self.results = results | ||
|
||
def _bing_request(self, endpoint: str, query: str, keys: List[str]): | ||
response = requests.get( | ||
ENDPOINT_BASE_URL + endpoint, | ||
headers={ 'Ocp-Apim-Subscription-Key': self.api_key }, | ||
params={ 'q': query, 'mkt': self.lang, 'count': self.results } | ||
) | ||
response_json = response.json() | ||
return [[result[key] for key in keys] for result in response_json['value']] | ||
|
||
|
||
def bing_news_search(self, query: str): | ||
""" | ||
Make a query to bing news search. Useful for finding news on a query. | ||
Args: | ||
query (str): The query to be passed to bing. | ||
""" | ||
return self._bing_request('news/search', query, ['name', 'description', 'url']) | ||
|
||
|
||
def bing_image_search(self, query: str): | ||
""" | ||
Make a query to bing images search. Useful for finding an image of a query. | ||
Args: | ||
query (str): The query to be passed to bing. | ||
returns a url of the images found | ||
""" | ||
|
||
return self._bing_request('images/search', query, ['name', 'contentUrl']) | ||
|
||
|
||
|
||
def bing_video_search(self, query: str): | ||
""" | ||
Make a query to bing video search. Useful for finding a video related to a query. | ||
Args: | ||
query (str): The query to be passed to bing. | ||
""" | ||
|
||
return self._bing_request('videos/search', query, ['name', 'contentUrl']) | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,152 @@ | ||
{ | ||
"cells": [ | ||
{ | ||
"cell_type": "code", | ||
"execution_count": 1, | ||
"id": "a2408d6e-8e07-47e5-a7e3-daf3022a44de", | ||
"metadata": {}, | ||
"outputs": [], | ||
"source": [ | ||
"# Setup OpenAI Agent\n", | ||
"import openai\n", | ||
"openai.api_key = 'sk-your-key'\n", | ||
"from llama_index.agent import OpenAIAgent\n" | ||
] | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"execution_count": 2, | ||
"id": "3e91e13e-d7da-47d1-8122-5e11bb1b5a5a", | ||
"metadata": {}, | ||
"outputs": [ | ||
{ | ||
"name": "stdout", | ||
"output_type": "stream", | ||
"text": [ | ||
"=== Calling Function ===\n", | ||
"Calling function: bing_news_search with args: {\n", | ||
" \"query\": \"latest news about superconductors\"\n", | ||
"}\n", | ||
"Got output: [['Scientists skeptical of superconductor claims that went viral', 'A recent claim by South Korean researchers that they have created a material which works as a superconductor at room temperature—long a holy grail of physics—has been met with huge excitement on social media but skepticism from scientists.', 'https://phys.org/news/2023-08-scientists-skeptical-superconductor-viral.html'], [\"Room-temperature superconductors: The facts behind the 'holy grail' of physics\", 'Onto this crowded scene comes LK-99, a material whose resistivity, its researchers claim, drops to near zero at 86 F (30C). The material is made up of mixed powders containing lead, oxygen, sulfur and phosphorus which is doped with copper. It is also relatively easy to manufacture and test.', 'https://www.msn.com/en-us/news/technology/room-temperature-superconductors-the-facts-behind-the-holy-grail-of-physics/ar-AA1eVoxP'], ['Scientists Sceptical Of Superconductor Claims That Went Viral', 'A recent claim by South Korean researchers that they have created a material which works as a superconductor at room temperature -- long a holy grail of physics -- has been met with huge excitement on social media but scepticism from scientists.', 'https://www.barrons.com/news/scientists-sceptical-of-superconductor-claims-that-went-viral-ee5e0918?refsec=topics_afp-news']]\n", | ||
"========================\n", | ||
"Here are some of the latest news articles about superconductors:\n", | ||
"\n", | ||
"1. \"Scientists skeptical of superconductor claims that went viral\" - This article discusses a recent claim by South Korean researchers that they have created a material which works as a superconductor at room temperature. The claim has generated excitement on social media but skepticism from scientists. [Read more](https://phys.org/news/2023-08-scientists-skeptical-superconductor-viral.html)\n", | ||
"\n", | ||
"2. \"Room-temperature superconductors: The facts behind the 'holy grail' of physics\" - This article provides information about LK-99, a material that claims to exhibit superconductivity at room temperature. The material is made up of mixed powders containing lead, oxygen, sulfur, and phosphorus, and it is relatively easy to manufacture and test. [Read more](https://www.msn.com/en-us/news/technology/room-temperature-superconductors-the-facts-behind-the-holy-grail-of-physics/ar-AA1eVoxP)\n", | ||
"\n", | ||
"3. \"Scientists Sceptical Of Superconductor Claims That Went Viral\" - This article also discusses the claim made by South Korean researchers about a room temperature superconductor. It highlights the excitement on social media but the skepticism from scientists. [Read more](https://www.barrons.com/news/scientists-sceptical-of-superconductor-claims-that-went-viral-ee5e0918?refsec=topics_afp-news)\n", | ||
"\n", | ||
"You can click on the links to read the full articles.\n" | ||
] | ||
} | ||
], | ||
"source": [ | ||
"from llama_hub.tools.bing_search.base import BingSearchToolSpec\n", | ||
"\n", | ||
"bing_tool = BingSearchToolSpec(\n", | ||
" api_key='your-key'\n", | ||
")\n", | ||
"agent = OpenAIAgent.from_tools(\n", | ||
" bing_tool.to_tool_list(),\n", | ||
" verbose=True,\n", | ||
")\n", | ||
"\n", | ||
"print(agent.chat('whats the latest news about superconductors'))" | ||
] | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"execution_count": 3, | ||
"id": "80a8981a-ba62-4c86-a755-a4ec26051805", | ||
"metadata": {}, | ||
"outputs": [ | ||
{ | ||
"name": "stdout", | ||
"output_type": "stream", | ||
"text": [ | ||
"=== Calling Function ===\n", | ||
"Calling function: bing_image_search with args: {\n", | ||
" \"query\": \"LK-99 superconductor\"\n", | ||
"}\n", | ||
"Got output: [['What is the LK-99 superconductor and why is it called the Holy Grail of ...', 'https://cdn.computerhoy.com/sites/navi.axelspringer.es/public/media/image/2023/08/superconductor-lk-99-3103376.jpg?tf=1200x'], ['The LK-99 controversy: between Nobel prospects and skeptical scrutiny ...', 'https://media.cybernews.com/images/featured-big/2023/08/LK-99-superconductor.png'], ['Will the LK-99 superconductor change the world?', 'https://images.moneycontrol.com/static-mcnews/2023/08/lk-99-superconductor-bbo-770x433.jpg?impolicy=website&width=770&height=431']]\n", | ||
"========================\n", | ||
"Here are some images of the LK-99 superconductor:\n", | ||
"\n", | ||
"1. 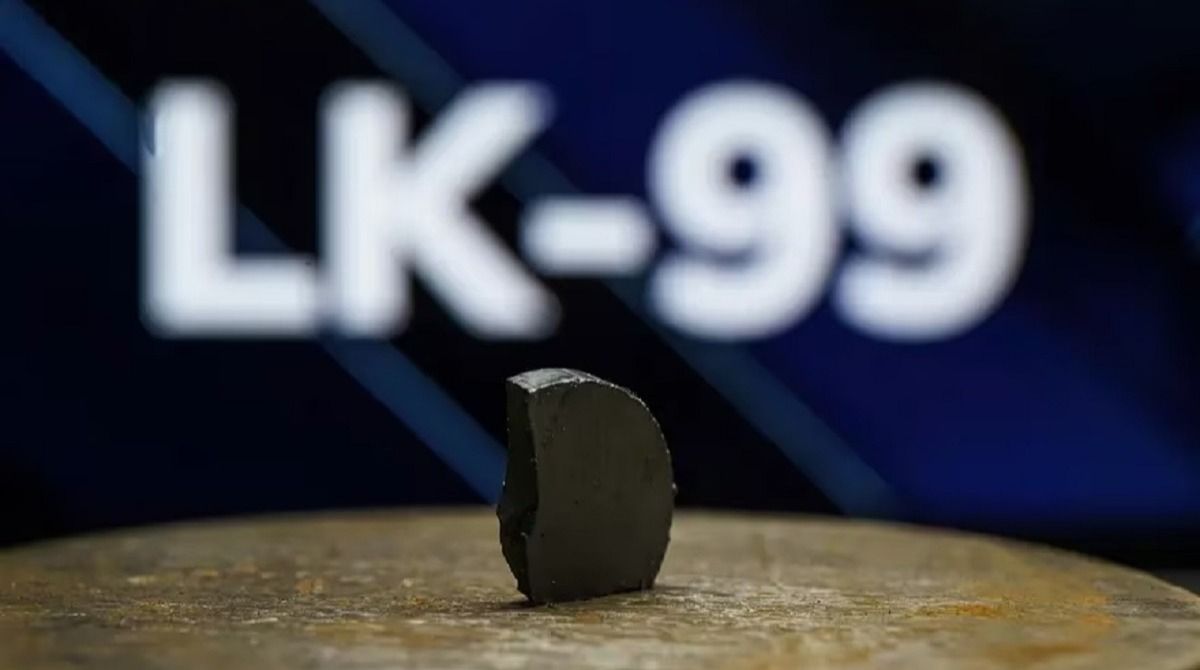\n", | ||
"\n", | ||
"2. \n", | ||
"\n", | ||
"3. 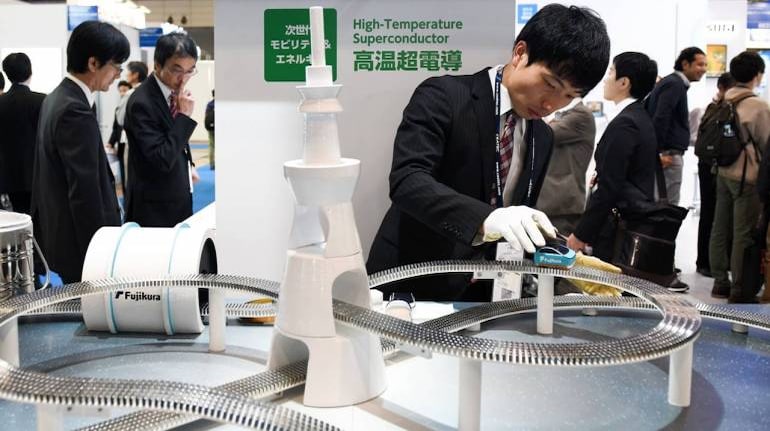\n", | ||
"\n", | ||
"Please note that these images are for illustrative purposes and may not represent the exact appearance of the LK-99 superconductor.\n" | ||
] | ||
} | ||
], | ||
"source": [ | ||
"print(agent.chat('what does lk-99 look like'))" | ||
] | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"execution_count": 4, | ||
"id": "4aa378d0-b797-4323-a91c-06baf19fe4b4", | ||
"metadata": {}, | ||
"outputs": [ | ||
{ | ||
"name": "stdout", | ||
"output_type": "stream", | ||
"text": [ | ||
"=== Calling Function ===\n", | ||
"Calling function: bing_video_search with args: {\n", | ||
" \"query\": \"LK-99 superconductor levitation\"\n", | ||
"}\n", | ||
"Got output: [['LK-99, a candidate for room temperature superconductivity', 'https://www.youtube.com/watch?v=AFDH4asSdqk'], ['「超電導体の磁気浮上を室温かつ常圧で確認した」とされるムービー【LK-99】', 'https://www.youtube.com/watch?v=aw5sw5TDihU'], ['Boaz Almog \"levitates\" a superconductor', 'https://www.youtube.com/watch?v=PXHczjOg06w']]\n", | ||
"========================\n", | ||
"Here are some videos of the LK-99 superconductor levitating:\n", | ||
"\n", | ||
"1. [LK-99, a candidate for room temperature superconductivity](https://www.youtube.com/watch?v=AFDH4asSdqk)\n", | ||
"\n", | ||
"2. [「超電導体の磁気浮上を室温かつ常圧で確認した」とされるムービー【LK-99】](https://www.youtube.com/watch?v=aw5sw5TDihU)\n", | ||
"\n", | ||
"3. [Boaz Almog \"levitates\" a superconductor](https://www.youtube.com/watch?v=PXHczjOg06w)\n", | ||
"\n", | ||
"You can click on the links to watch the videos.\n" | ||
] | ||
} | ||
], | ||
"source": [ | ||
"print(agent.chat('is there any videos of it levitating'))" | ||
] | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"execution_count": null, | ||
"id": "56ca5c22-0eab-42ec-b038-7acb9cab710c", | ||
"metadata": {}, | ||
"outputs": [], | ||
"source": [] | ||
} | ||
], | ||
"metadata": { | ||
"kernelspec": { | ||
"display_name": "Python 3 (ipykernel)", | ||
"language": "python", | ||
"name": "python3" | ||
}, | ||
"language_info": { | ||
"codemirror_mode": { | ||
"name": "ipython", | ||
"version": 3 | ||
}, | ||
"file_extension": ".py", | ||
"mimetype": "text/x-python", | ||
"name": "python", | ||
"nbconvert_exporter": "python", | ||
"pygments_lexer": "ipython3", | ||
"version": "3.9.12" | ||
} | ||
}, | ||
"nbformat": 4, | ||
"nbformat_minor": 5 | ||
} |