-
Notifications
You must be signed in to change notification settings - Fork 0
Spring Boot 1.4.0 M2 Release Notes
For changes in earlier milestones, please refer to:
See instructions in the 1.4.0.M1 release notes for upgrading from v1.3.
Spring Boot 1.4 ships with a new spring-boot-test
module that contains a completely reorganized org.springframework.boot.test
package. When upgrading a Spring Boot 1.3 application you should migrate from the deprecated classes in the old package to the equivalent class in the new structure. If you’re using Linux or OSX, you can use the following command to migrate code:
find . -type f -name '*.java' -exec sed -i '' \ -e s/org.springframework.boot.test.ConfigFileApplicationContextInitializer/org.springframework.boot.test.context.ConfigFileApplicationContextInitializer/g \ -e s/org.springframework.boot.test.EnvironmentTestUtils/org.springframework.boot.test.util.EnvironmentTestUtils/g \ -e s/org.springframework.boot.test.OutputCapture/org.springframework.boot.test.rule.OutputCapture/g \ -e s/org.springframework.boot.test.SpringApplicationContextLoader/org.springframework.boot.test.context.SpringApplicationContextLoader/g \ -e s/org.springframework.boot.test.SpringBootMockServletContext/org.springframework.boot.test.mock.web.SpringBootMockServletContext/g \ -e s/org.springframework.boot.test.TestRestTemplate/org.springframework.boot.test.web.client.TestRestTemplate/g \ {} \;
Additionally, Spring Boot 1.4 attempts to rationalize and simplify the various ways that a Spring Boot test can be run. You should migrate the following to use the new @SpringBootTest
annotation:
-
From
@SpringApplicationConfiguration(classes=MyConfig.class)
to@SpringBootTest(classes=MyConfig.class)
-
From
@ContextConfiguration(classes=MyConfig.class, loader=SpringApplicationContextLoader.class)
to@SpringBootTest(classes=MyConfig.class)
-
From
@ContextConfiguration(classes=MyConfig.class, loader=SpringApplicationContextLoader.class)
to@SpringBootTest(classes=MyConfig.class)
-
From
@IntegrationTest
to@SpringBootTest(webEnvironment=WebEnvironment.NONE)
-
From
@IntegrationTest with @WebAppConfiguration
to@SpringBootTest(webEnvironment=WebEnvironment.DEFINED_PORT)
(orRANDOM_PORT
) -
From
@WebIntegrationTest
to@SpringBootTest(webEnvironment=WebEnvironment.DEFINED_PORT)
(orRANDOM_PORT
)
Tip
|
Whilst migrating tests you may also want to replace any @RunWith(SpringJUnit4ClassRunner.class) declarations with Spring 4.3’s more readable @RunWith(SpringRunner.class) .
|
For more details on the @SpringBootTest
annotation refer to the updated documentation.
Hibernate 5.1 is now used as the default JPA persistence provider. If you are upgrading from Spring Boot 1.3 you will be moving from Hibernate 4.3 to Hibernate 5.1. Please refer to Hibernate migration documentation for general upgrade instructions. In addition you should be aware of the following:
-
SpringNamingStrategy
is no longer used as Hibernate 5.1 has removed support for the oldNamingStrategy
interface. A newSpringPhysicalNamingStrategy
is now auto-configured which is used in combination with Hibernate’s defaultImplicitNamingStrategy
. This should be very close to (if not identical) to Spring Boot 1.3 defaults, however, you should check your Database schema is correct when upgrading. -
In order to minimize upgrade pain, we set
hibernate.id.new_generator_mappings
is set tofalse
for Hibernate 5. The Hibernate team generally don’t recommend this setting, so if your happy to deal with generator changes, you might want to setspring.jpa.hibernate.use-new-id-generator-mappings
totrue
in yourapplication.properties
file.
If you have major problems upgrading to Hibernate 5.1 you can downgrade to the older Hibernate version by overriding the hibernate.version
property in your pom.xml
:
<properties>
<hibernate.version>4.3.11.Final</hibernate.version>
</properties>
Note
|
Hibernate 4.3 will not be supported past Spring Boot 1.4. Please raise an issue if you find a bug that prevents you from upgrading. |
Spring Boot 1.4 builds on and requires Spring Framework 4.3. There are a number of nice refinements in Spring Framework 4.3 including new Spring MVC @RequestMapping
annotations, Refer to the Spring Framework reference documentation for details.
A number of third party libraries have been upgraded to the latest version. Highlights include Hibernate 5.1, Jackson 2.7, Solr 5.5, Spring Data Hopper, Spring Session 1.2 & Hazelcast 3.6.
You can now use image files to render ASCII art banners. Drop a banner.gif
, banner.jpg
or banner.png
file into src/main/resources
to have it automatically converted to ASCII. You can use the banner.image.width
and banner.image.height
properties to tweak the size, or use banner.image.invert
to invert the colors.
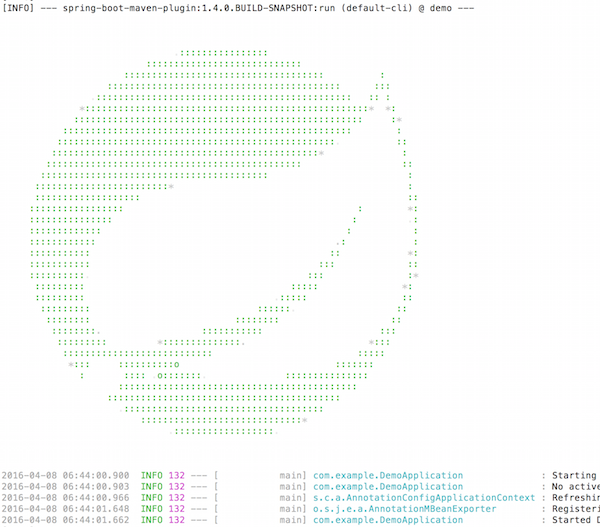
A new @JsonComponent
annotation is now provided for custom Jackson JsonSerializer
and/or JsonDeserializer
registration. This can be a useful way to decouple JSON serialization logic:
@JsonComponent
public class Example {
public static class Serializer extends JsonSerializer<SomeObject> {
// ...
}
public static class Deserializer extends JsonDeserializer<SomeObject> {
// ...
}
}
Additionally, Spring Boot also now provides JsonObjectSerializer
and JsonObjectDeserializer
base classes which provide useful alternatives to the standard Jackson versions when serializing Objects. See the updated documentation for details.
Full auto-configuration support is now provided for Couchbase. You can easily access a Bucket
and Cluster bean by adding the `spring-boot-starter-data-couchbase
starter POM and providing a little configuration:
spring.couchbase.bootstrap-hosts=my-host-1,192.168.1.123 spring.couchbase.bucket.name=my-bucket spring.couchbase.bucket.password=secret
It’s also possible to use Couchbase as a backing store for a Spring Data Repository
or as a CacheManager
. Refer to the updated documentation for details.
Auto-configuration support is now provided for Neo4J. You can connect to a remote server or run an embedded Neo4J server. You can also use Neo4J to back a Spring Data Repository
, for example:
public interface CityRepository extends GraphRepository<City> {
Page<City> findAll(Pageable pageable);
City findByNameAndCountry(String name, String country);
}
Full details are provided in the Neo4J section of the reference documentation.
Auto-configuration support is now included for the Narayana transaction manager. You can choose between Narayana, Bitronix or Atomkos if you need JTA support. See the updated reference guide for details.
You can now use the InfoContributor
interface to register beans that expose information to the /info
actuator endpoint. Out of the box support is provided for:
-
Full or partial Git information generated from a build plugin (set
management.info.git.mode=full
to expose full details) -
Build information generated from the Spring Boot Maven or Gradle plugin.
-
Custom information from the Environment (any property starting
info.*
)
Details are documented in the "Application information" section of the reference docs.