We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
将O(n^3) 复杂度转换成 O(n) 分层求异,tree diff 相同类生成相似树形结构,不同类生成不同树形结构,component diff 设置唯一 key ,对 element diff 进行算法优化 只比较同一层级,不跨级比较;tag 不同,则直接删掉重建,不再深度比较;tag 和 key 两者都相同,则认为是相同节点,不再深度比较
react diff 和 vue diff 的区别
jsx 经过 babel 转换成 React.createElement,createElement 参数有 type: 标签类型,attributes: 属性,剩下的是子元素 createElement 将处理成 type props: { className: '', children: [] } 称之为 element 对象 element 不一定是 Object 类型
ReactDOMComponent、ReactCompositeComponentWrapper 是 React 的私有类,他们常用的方法有 mountComponent、updateComponent,生命周期和 render 函数都是在这些私有类的方法里被调用的
首次渲染
实例化类,调用类的 render 方法拿到渲染的元素,调用 mountComponent 方法将元素挂载到 dom 上,递归处理
渲染更新
state 改变,将 state 放入 pendingState 中存起来,再判断是否处于批量更新阶段,如果是,将当期组件的实例放进 dirtyComponent 中,收集完毕后,遍历 dirtyComponent 数组,调用 updateComponent 对组件进行更新
事件的 event 是 合成事件(SyntheicEvent,模拟出来 DOM 事件所有能力),不是原生事件,event.nativeEvent.target 触发是 button,event.nativeEvent.currentTarget 绑定是 document,event.nativeEvent 是原生对象。所以说 react 事件都挂载在 document 上
Vue 触发和绑定都是 button,event 也是原生事件,和 dom 事件一样 为什么要用合成事件机制?
react 15 及以前
react 16.4 以后:https://projects.wojtekmaj.pl/react-lifecycle-methods-diagram/
getDerivedStatefromProps: 在 render 之前调用,并且在初始挂载及后续更新时都会被调用,返回一个新的对象来更新 state,返回 null 则不更新。像 componentWillReceiveProps
getSnapshotBeforeUpdate: 在 componentDidUpdate 之前调用,使组件能够在发生改变之前从dom中捕获一些信息,比如 滚动位置
useState useReducer useEffect(useEffect 完整指南) useRef useCallback useMemo
是基于浏览器的单线程调度算法,React 16 之前,reconciliation 算法是递归,无法中断,fiber 将递归拆成无数个小任务去执行,随时能够停止、恢复,时机取决于当前的一帧(16ms)内,是否有足够的时间允许计算。
protals context 异步组件:import() + React.lazy + React.Suspense HOC render props
受控组件:受 state 控制 非受控组件:不受 state 控制,用 ref 拿值
props context redux setState 同步的情况 自定义事件中,setState 是同步的; setTimeout 中,setState 是同步的
shouldComponentUpdate PureComponent 和 React.memo 不可变值 immutable.js
setState 主流程:newState 存入 pending 队列,判断当前是否处于 batch update(isBatchingUpdates),是,保存组件于 dirtyComponents 中,否,遍历所有的 dirtyComponents,调用 updateComponent,更新 pending state or props
哪些能命中 batchUpdate 机制,即 isBatchingUpdates 判断为 true?
不能命中的有:
函数式组件捕获了渲染所使用的值
How Are Function Components Different from Classes?
The text was updated successfully, but these errors were encountered:
No branches or pull requests
diff原理
将O(n^3) 复杂度转换成 O(n)
分层求异,tree diff
相同类生成相似树形结构,不同类生成不同树形结构,component diff
设置唯一 key ,对 element diff 进行算法优化
只比较同一层级,不跨级比较;tag 不同,则直接删掉重建,不再深度比较;tag 和 key 两者都相同,则认为是相同节点,不再深度比较
react diff 和 vue diff 的区别
渲染原理
jsx 经过 babel 转换成 React.createElement,createElement 参数有 type: 标签类型,attributes: 属性,剩下的是子元素
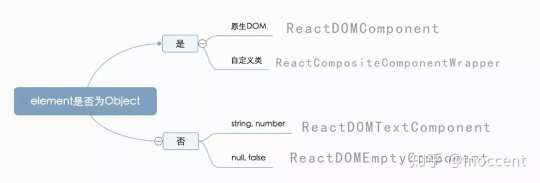
createElement 将处理成 type props: { className: '', children: [] } 称之为 element 对象
element 不一定是 Object 类型
ReactDOMComponent、ReactCompositeComponentWrapper 是 React 的私有类,他们常用的方法有 mountComponent、updateComponent,生命周期和 render 函数都是在这些私有类的方法里被调用的
首次渲染
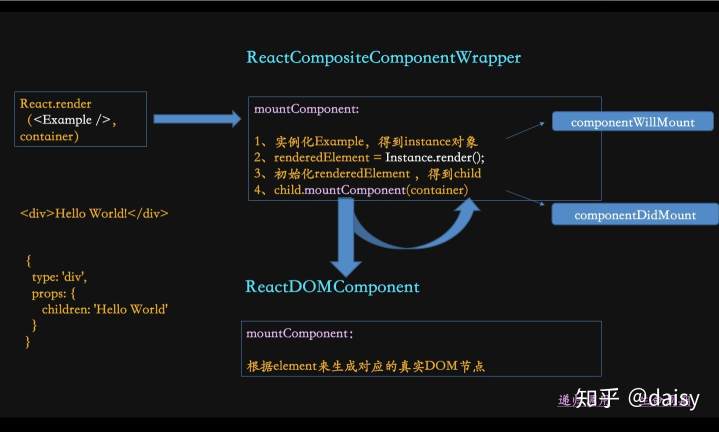
实例化类,调用类的 render 方法拿到渲染的元素,调用 mountComponent 方法将元素挂载到 dom 上,递归处理
渲染更新
state 改变,将 state 放入 pendingState 中存起来,再判断是否处于批量更新阶段,如果是,将当期组件的实例放进 dirtyComponent 中,收集完毕后,遍历 dirtyComponent 数组,调用 updateComponent 对组件进行更新
事件机制
事件的 event 是 合成事件(SyntheicEvent,模拟出来 DOM 事件所有能力),不是原生事件,event.nativeEvent.target 触发是 button,event.nativeEvent.currentTarget 绑定是 document,event.nativeEvent 是原生对象。所以说 react 事件都挂载在 document 上
Vue 触发和绑定都是 button,event 也是原生事件,和 dom 事件一样
为什么要用合成事件机制?
组件生命周期
react 15 及以前
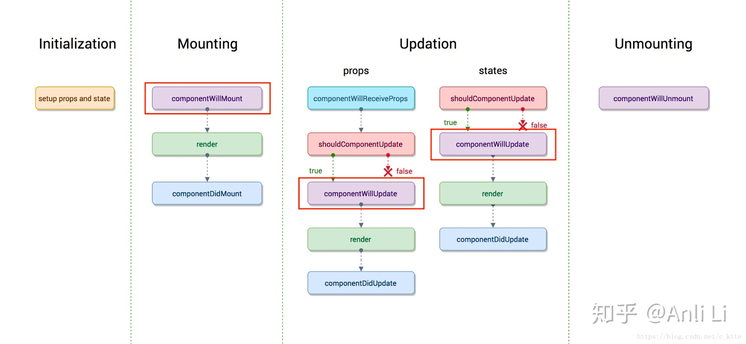
react 16.4 以后:https://projects.wojtekmaj.pl/react-lifecycle-methods-diagram/
getDerivedStatefromProps: 在 render 之前调用,并且在初始挂载及后续更新时都会被调用,返回一个新的对象来更新 state,返回 null 则不更新。像 componentWillReceiveProps
getSnapshotBeforeUpdate: 在 componentDidUpdate 之前调用,使组件能够在发生改变之前从dom中捕获一些信息,比如 滚动位置
react hooks
useState
useReducer
useEffect(useEffect 完整指南)
useRef
useCallback
useMemo
Fiber
是基于浏览器的单线程调度算法,React 16 之前,reconciliation 算法是递归,无法中断,fiber 将递归拆成无数个小任务去执行,随时能够停止、恢复,时机取决于当前的一帧(16ms)内,是否有足够的时间允许计算。
react 高级特性
protals
context
异步组件:import() + React.lazy + React.Suspense
HOC
render props
受控组件和非受控组件
受控组件:受 state 控制
非受控组件:不受 state 控制,用 ref 拿值
组件通信
props
context
redux
setState 同步的情况
自定义事件中,setState 是同步的;
setTimeout 中,setState 是同步的
React 性能优化
shouldComponentUpdate
PureComponent 和 React.memo
不可变值 immutable.js
React batchUpdate 机制
setState 主流程:newState 存入 pending 队列,判断当前是否处于 batch update(isBatchingUpdates),是,保存组件于 dirtyComponents 中,否,遍历所有的 dirtyComponents,调用 updateComponent,更新 pending state or props
哪些能命中 batchUpdate 机制,即 isBatchingUpdates 判断为 true?
不能命中的有:
函数组件和类组件的区别
函数式组件捕获了渲染所使用的值
How Are Function Components Different from Classes?
Redux
transaction 事务机制
The text was updated successfully, but these errors were encountered: